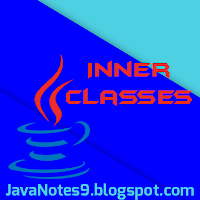
Inner classes :
To define one class into another, this process is called as inner classes.There are four type of inner classes
1) Member inner class.
2) Static inner class.
3) Local inner class.
4) Anonymous inner class.
Member inner class :
A class which is defined as a member of some outer class is called as member inner class.Write a program to define the member in inner class.
class outer { int x=10; void fun1() { System.out.println("Hello outer class method"); } class inner { int y=20; void fun2() { System.out.println("Hello inner class method"); } } //inner class end public static void main(String args[]) { outer a1=new outer(); System.out.println("a1.x"); a1.fun1(); //a1.fun2();error //Inner il=new Inner();error outer.Inner i2=new Outer().new Inner(); System.out.println(i2.y); //il.fun1();error } } //outer class end
Static inner class :
Inner classes can be static we can instantiate this class directly or by the outer class name.Write a program to define the static inner class.
class outer { static int x=10; void fun1() { System.out.println("Hello outer class method"); } static class inner { int y=20; void fun2() { System.out.println("Hello inner class method"); } } //inner class end public static void main(String args[]) { outer a1=new outer(); System.out.println("X is "+x); System.out.println("X is "+a1.x); a1.fun1(); Inner i=new Inner(); System.out.println("Y is "+i.y); i.fun2(); // OR outer.Inner il=new outer.inner(); System.out.println("Y is "+il.y); il.fun2(); } } //outer class end
Local inner class :
A class which is defined in method body of outer class, this is called as local inner class. The scope and accessibility of this class is limited up to the method in which it is defined.Write a program to define the local inner class.
class outer1 { int y=20; void fun1() { System.out.println("Enter into method"); class local { int x=50; void fun2() { System.out.println("Hello local class method"); } }; local L1=new local(); System.out.println("X is "+L1.x); L1.fun2(); System.out.println("Exit from method"); } //fun1 public static void main(String args[]) { outer1 a1=new outer1(); System.out.println("Y is "+a1.y); a1.fun1(); } } //outer class end
Anonymous inner class :
A local inner class, which does not have name and implements the outside interface, is called as anonymous inner class.Write a program to define the anonymous inner class.
interface xyz { public void fun1(); public void fun2(); } class test { public xyz getxyz1() { xyz x1=new xyz() { public void fun1() { System.out.println("Hello first class fun1"); } public void fun2() { System.out.println("Hello first class fun2"); } }; return(x1); } //getxyz1 public xyz getxyz2() { xyz x2= new xyz() { public void fun1() { System.out.println("Hello second class fun1"); } public void fun2() { System.out.println("Hello second class fun2"); } }; return(x2); } //getxyz2 public static void main(String args[]) { Test t1=new Test(); xyz x3=t1.getxyz1(); xyz x4=t1.getxyz2(); x3.fun1(); x3.fun2(); x4.fun1(); x4.fun2(); } }
class AB { { System.out.println("Non static block"); } static { System.out.println("static block"); } AB() { System.out.println("Constructor block"); } fun1() { System.out.println("Method block"); } public static void main(String args[]) { System.out.println("Main block"); AB a1=new AB(); a1.fun1(); AB a2=new AB(); } }
static block
Main block
Constructor block
Method block
Non static block
constructor block
Post A Comment: